GetObjectSpellOwner(obj) -- if the object is a spell (missile), it will return an object of it's creator/caster
GetObjectSpellStartPos(obj) -- if the object is a spell (missile), it returns XYZ of the starting cast position
GetObjectSpellEndPos(obj) -- if the object is a spell (missile), it returns XYZ of the ending cast position
GetObjectSpellPlacementPos(obj) -- if the object is a spell (missile), it returns XYZ of the mouse placement cast position
GetObjectSpellTarget(obj) -- if the object is a spell (missile), and the spell has a target, returns an object of the target
GetObjectOwner(obj) -- if the object is a ward or trap or anything like that, this function returns an object pointer of it's owner
GetCurrentGold(obj) -- (probably works only with YOUR hero), returns the current gold you have
GetTotalEarnedGold(obj) -- (probably works only with YOUR hero), returns the total gold earned since the start of the game
Some code examples:
local savedobject = nil OnCreateObj(function(Object) --Object is a pointer to the object created by the game just now. if Object ~= nil then if GetObjectBaseName(Object) == 'missile' then --check if it's missile savedobject = Object --store the object somewhere for later use end end end) OnDraw(function(myHero) --myHero is a pointer to our champion; if savedobject ~= nil then --check if we have recently saved object if GetObjectSpellName(savedobject) == 'RyzeQ' then --check if it's Ryze Q spell caster = GetObjectSpellOwner(savedobject); --grab the owner (caster) of the RyzeQ if caster ~= nil then DrawCircle(GetObjectSpellStartPos(savedobject),100,0,0,ARGB(255,255,0,0)); --draw starting pos DrawCircle(GetObjectSpellEndPos(savedobject),100,0,0,ARGB(255,0,255,0)); --draw ending pos DrawCircle(GetObjectSpellPlacementPos(savedobject),100,0,0,ARGB(255,0,0,255)); --draw mouse placement pos DrawCircle(GetOrigin(savedobject),100,0,0,ARGB(255,255,255,255)); --draw current pos DrawText(GetObjectName(caster), 30,600,200,ARGB(255,255,255,0)) --draw the name of caster DrawText(GetCurrentGold(caster), 30,600,250,ARGB(255,255,255,0)) --draw how much gold the caster has right now DrawText(GetTotalEarnedGold(caster), 30,600,300,ARGB(255,255,255,0)) --draw the total gold earned by the caster from the start end end end end)
How this script looks like in-game:
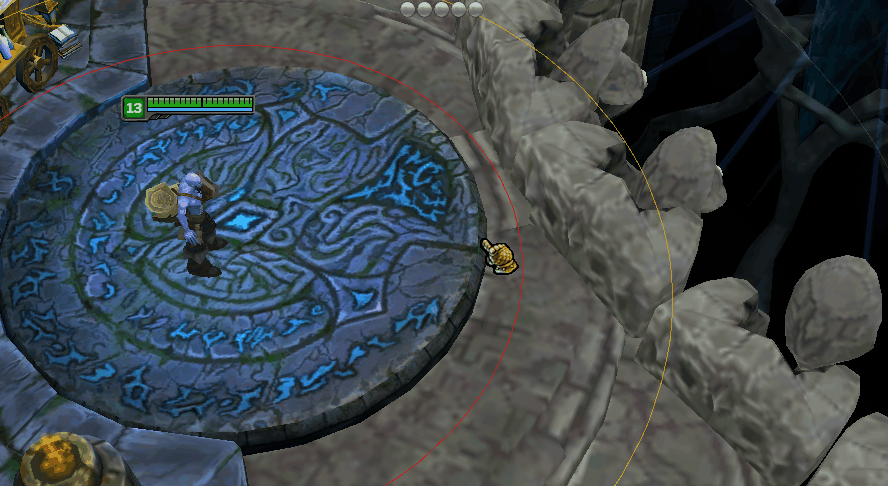